
I won’t dig into the rules around mutable references here, but know that there are some gotchas! Rust prevents data races let mut s = String :: from ( "a regular string" ) let other_s = & mut s But they can be with a special keyword: mut. Neat, huh? but can I mutate that reference? Since we passed a reference (the & is the key)to calculate_length, and the function does not own it (it just refers to the value), s1 won’t be dropped when that reference goes out of scope in calculate_length. Here’s an example of a reference: let s1 = String :: from ( "balling" ) let len = calculate_length ( &s1 ) Sometimes, you don’t want that value to be dropped though. “dropping dimes” means “to make an assist in basketball” (source: Urban Dictionary #3) But they’re close, right?ĭropping values is something Rust will do for you (assist?). If not it will be moved.Īnd recommended this StackOverflow answer as a resource to dig deeper. Still working through understanding this.Įssentially, if a struct implements the Copy trait it will be copied. But x is an i32 and gets copied instead of moved. s is a String type and the ownership is transferred to the function. The only difference I see is in the type of the variable being passed to each. But because s's value was moved, nothing // special happens. 64) : // Here, x goes out of scope, then s.
#RUST LANGUAGE BOOK CODE#
Here’s some code from Variable Scope section of the book (pg. The way it does this is by calling drop automatically at the closing curly bracket where your value goes out of scope. Here! A gift for you-it’s the memory that is no longer in use. The compiler does the work for you, no garbage collection needed! Instead of having to keep track of what memory you use throughout your program, Rust returns the memory after your variable goes out of scope. That’s all I’ll say here for now.įurther reading: What’s the difference? - String::from() vs “literal” Rust won’t let your memory go to waste The key here is that "hello" is a string literal. It takes in a string literal like "hello" and returns a String. String is a string type which has a function under its namespace called from(). I won’t go into depth on this because I could write an entire article on it, but look at this code: let s = String :: from ( "hello" ) As they say in the book,Īt this point, the relationship between scopes and when variables are valid is similar to that in other programming languages. Values are only valid within a certain range.

In JavaScript, there are rules where variables are only accessible within specific scopes. But be aware that by dropping a value when it goes out of scope, we remove the ability to use or reference it. You can’t remove memory though.ĭropping seems to be the one the authors use so we will stick with that.
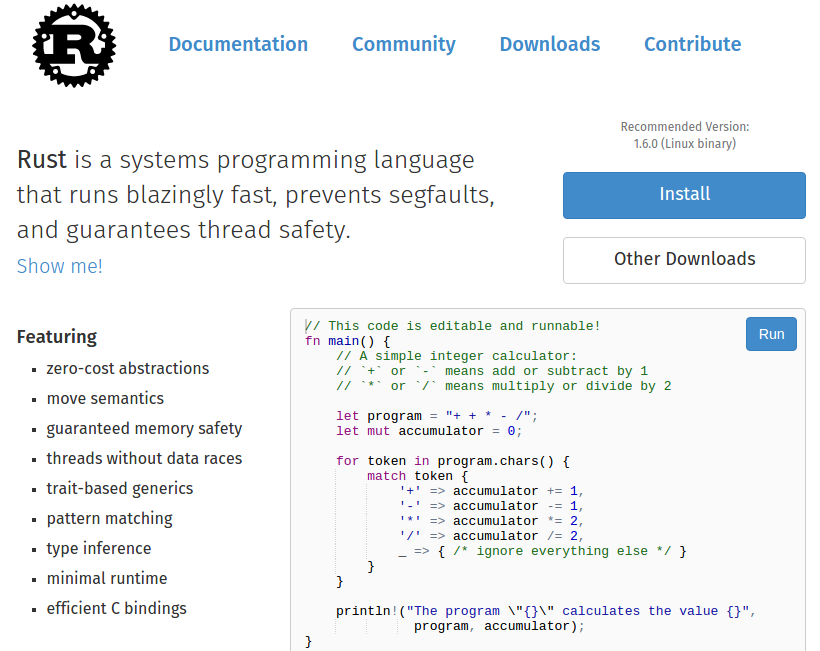
I don’t want to hyper-focus because it isn’t useful. Removing can mean the same thing depending on how you use it. Dropping a value in the context of ownership means that the value is no longer guaranteed to be in memory. I asked myself this question, and I think the answer is it depends. is dropping a value the same as removing a value?
#RUST LANGUAGE BOOK FREE#
This means that Rust can guarantee a value is never owned by more than one owner so that it can safely drop the value when it’s out of scope and free up that memory.
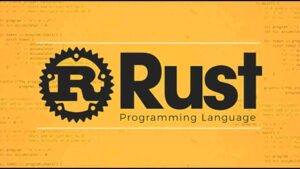
#RUST LANGUAGE BOOK SERIES#
This is the next blog post in a series related to The Rust Programming Language Book.
